How to allow a child component to access and modify a parent's state in React
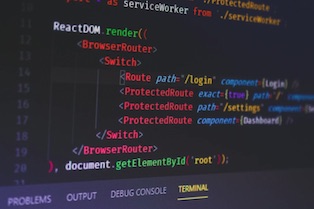
Let’s say your React application has a smart component (i.e. the component has its own state), and you want a child component to be able to not only access, but actually modify that parent component’s state.
How can you give a child component this functionality?
- Define this state-modifying function in the parent component.
- Pass this function to the child component as a prop.
- Have a trigger inside the child component that calls this function
Here’s an example.
We have an App
component, which has a state that includes blogs
(an array), and searchfield
(a string).
We want a child component, SearchBox
, to be able to update the searchfield
string.
1. Define the function in the parent component
Our first step is to define a function, onSearchChange
, inside the App
class definition.
This function takes an event as an argument, and updates searchfield
with the target value of that event.
class App extends React.Component {
constructor() {
super();
this.state = {
blogs: [],
searchfield: "",
};
}
onSearchChange = (event) => {
this.setState({ searchfield: event.target.value });
};
}
2. Pass this function as a prop
Next, still inside the App
component, we need to pass onSearchChange
as a prop when we render our child component SearchBox
.
render(){
return (
<div className = 'tc'>
<h1>My blog posts</h1>
<SearchBox onSearchChange={this.onSearchChange} />
<BlogDisplay blogs= { filteredBlogs } />
</div>
)
}
3. Include a trigger inside the child component that calls this function
Finally, in our SearchBox
component definition, we need to make sure we receive onSearchChange
as a prop, and then we need to call it when appropriate.
This is our entire SearchBox
component:
import React from "react";
const SearchBox = ({ onSearchChange }) => {
return (
<div className="pa2">
<input
type="search"
placeholder="search blogs"
onChange={onSearchChange}
/>
</div>
);
};
export default SearchBox;
Notice in the above that we used object destructuring to retrieve the onSearchChange
key from the props
object.
We could have done the following too, which does the exact same thing:
import React from "react";
const SearchBox = (props) => {
return (
<div className="pa2">
<input
type="search"
placeholder="search blogs"
onChange={props.onSearchChange}
/>
</div>
);
};
export default SearchBox;