How To Sort Array Elements in Javascript and Ruby
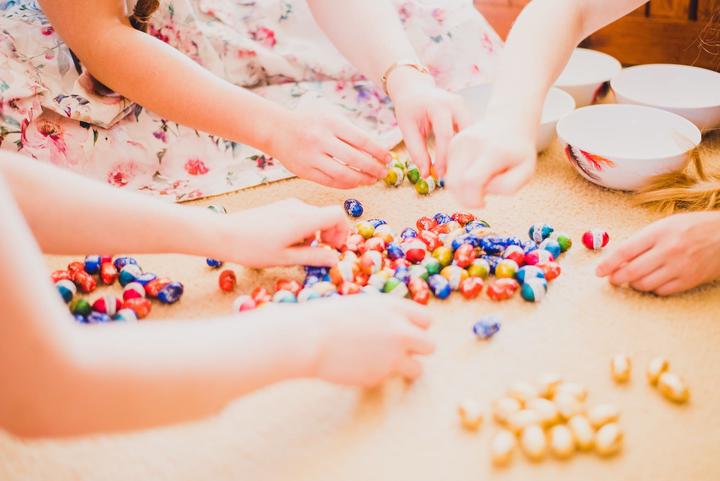
Sorting an array is one of the most common things we have to do as software engineers. It can also sometimes be the fastest way to calculate a data point–for example, the highest possible product of any three numbers in an array.
The most standard method to use in both Javascript and Ruby is the .sort
method. However, as we will see next, the name of the method is where the similarities between the two languages end.
Javascript
In Javascript, the .sort
method mutates the original array. So, if you need to keep the original array for whatever reason, you better only sort a copy of the original array.
Let’s look at the following code:
const arr = [1,4,-10,-200,-3,30,42,5,-1500,3];
arr.sort();
console.log(arr);
–> which outputs:
[ -10, -1500, -200, -3, 1, 3, 30, 4, 42, 5]
Several things to point out here:
- Even if we replaced line 2 with
const newArray = arr.sort()
, we’d get the same output (arr
is still mutated). - Notice that we were able to sort the array even though we declared it with
const
and notlet
. That’s because althoughconst
prevents us from pointingarr
to a new place in memory (i.e. using the=
symbol), it still allows us to rearrange the internal elements. Similarly, we could still use.push()
,.pop()
, etc, on an array declared withconst
. - Notice that
30
came before4
, and42
came before5
. What gives? And what’s going on with the negative numbers–shouldn’t they be: -1500, -200, -10, and -3? When you don’t add parameters to the.sort()
method, it treats each digit kind of like the way a dictionary treats each letter in a word. So, just like “gone” comes after “go” but before “hi”, so too does “42” come after “4” but before “5”. The same thing is happening to the negative numbers.
If we want to correctly sort by numerical value, we need to insert a function as a parameter in the .sort()
method.
- To sort in ascending order, we’d use:
arr.sort((a,b) => a-b);
- To sort in descending order, we’d use:
arr.sort((a,b) => b-a);
The ascending order sort would output:
[ -1500, -200, -10, -3, 1, 3, 4, 5, 30, 42]
The descending order sort would output the exact reverse.
Ruby
Let’s now look at how to do the same thing in Ruby. Here, the default .sort
method does not mutate the original array.
Let’s look at the following code:
arr = [1,4,-10,-200,-3,30,42,5,-1500,3]
arr.sort
print arr
–> which outputs:
[1,4,-10,-200,-3,30,42,5,-1500,3]
If we want to mutate the original array, we need to use .sort!
instead of .sort
:
arr = [1,4,-10,-200,-3,30,42,5,-1500,3]
arr.sort!
print arr
–> which outputs:
[-1500, -200, -10, -3, 1, 3, 4, 5, 30, 42]
Several things to point out here:
- Notice that, unlike in Javascript, the default
.sort
method in Ruby sorted by numerical value instead of by digit order. The default method does the exact same thing as this more defined sort:arr.sort { |a, b| a <=> b }
- If we wanted to sort in descending order, we can use:
arr.sort { |a, b| b <=> a }
- To do more complex sorts, check out the
.sort_by
method. This guide by Andrew Allen is good.